Securing RESTful APIs with OAuth 2.0 and Spring Security
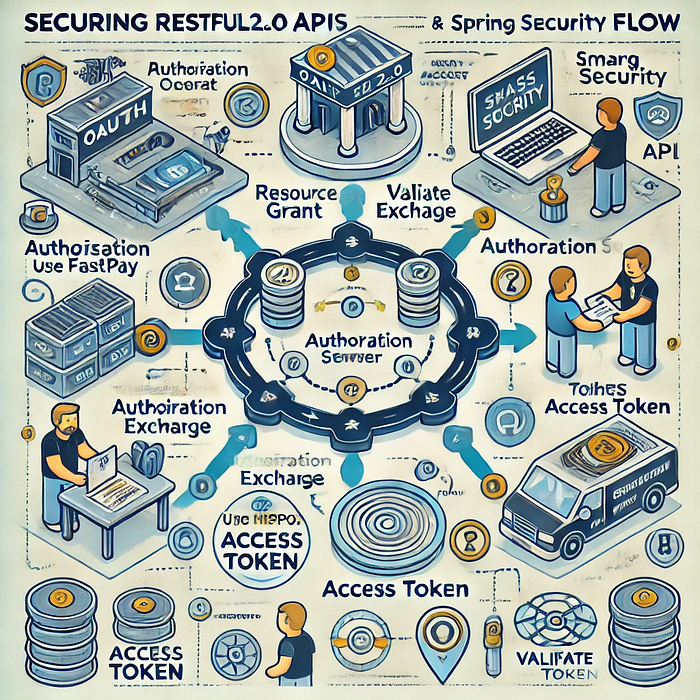
Views: 5
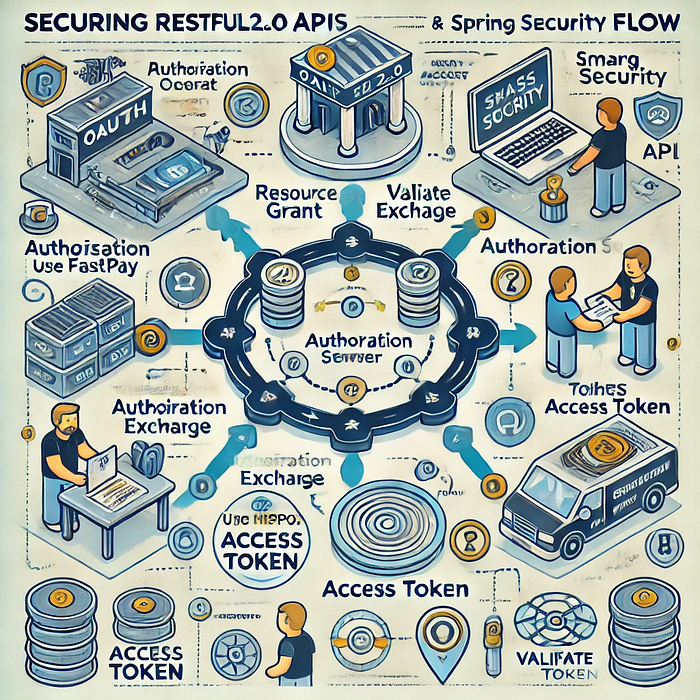
API security is essential in protecting sensitive data and ensuring authorized access. In this guide, we’ll explore:
- Technical implementation of OAuth 2.0 in Spring Security.
- Best practices for API security.
- A relatable real-life scenario to make the concepts easier to grasp.
As APIs power more applications, the need for robust security grows. OAuth 2.0, combined with Spring Security, offers a scalable solution for securing RESTful APIs. This blog dives deep into the technical details while illustrating the process with a real-life scenario.
Understanding OAuth 2.0: A Primer
OAuth 2.0 is a widely used authorization framework that provides secure, token-based access to resources.
Key Components
- Resource Owner: The individual or entity that owns the data (e.g., a user).
- Client: The application requesting access (e.g., a third-party app).
- Authorization Server: Issues tokens after validating credentials.
- Resource Server: Validates tokens and serves protected resources.
A Real-Life Scenario: Bob and the Grocery Store
Imagine Bob using an online grocery app, SmartGrocer, to order groceries and pay via FastPay. Here’s how OAuth 2.0 facilitates this process securely:
- Bob (Resource Owner)Â logs in to FastPay and authorizes SmartGrocer to access his payment account.
- SmartGrocer (Client)Â makes an authorization request to FastPay.
- FastPay (Authorization Server)Â issues an access token.
- SmartGrocer API (Resource Server)Â validates the token and processes the payment.
OAuth 2.0 in Action: Technical Walkthrough
1. Setting Up OAuth 2.0 in a Spring Boot Application
Start by adding dependencies for OAuth 2.0 and Spring Security in your project:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-oauth2-resource-server</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
2. Configuring Spring Security
AÂ SecurityConfig
 class to secure your REST API:
import org.springframework.context.annotation.Bean;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.web.SecurityFilterChain;
@Configuration
public class SecurityConfig {
@Bean
public SecurityFilterChain securityFilterChain(HttpSecurity http) throws Exception {
http
.authorizeHttpRequests(authorize -> authorize
.requestMatchers("/api/public/**").permitAll()
.anyRequest().authenticated()
)
.oauth2ResourceServer(oauth2 -> oauth2.jwt());
return http.build();
}
}
This setup:
- Secures endpoints by requiring authentication.
- Uses JWTs (JSON Web Tokens) for token-based access.
3. Connecting to an Authorization Server
Configure your application to use an external authorization server like Auth0 or Keycloak:
spring:
security:
oauth2:
resourceserver:
jwt:
issuer-uri: https://your-auth-server.com/
Mapping Bob’s Scenario to the Technical Process
- Authorization Request: Bob is redirected to FastPay to log in and approve SmartGrocer’s access.
- Token Exchange: SmartGrocer securely obtains an access token from FastPay.
- API Request: SmartGrocer uses the token to access protected resources (e.g., process payment).
Best Practices for Securing APIs
- Use HTTPS: Encrypt communication to prevent data interception.
- Validate Tokens: Always validate JWTs or tokens on the server side.
- Define Scopes and Roles: Limit token permissions for enhanced security.
- Rotate and Expire Tokens: Mitigate risks by implementing expiration and refresh policies.
- Implement Rate Limiting: Use tools like Spring Cloud Gateway to control API usage.
- Log and Monitor: Track suspicious activities and errors.
Benefits of Using Spring Security with OAuth 2.0
- Ease of Integration: Spring Security seamlessly integrates with Spring Boot applications.
- Scalability: Adaptable for both small-scale and enterprise-level applications.
- Flexibility: Allows custom authentication and authorization logic.
Visualizing the Process
Below is a professional diagram illustrating the OAuth 2.0 flow:
- Bob (Resource Owner)Â initiates a payment request on SmartGrocer.
- SmartGrocer redirects Bob to FastPay (Authorization Server) for login.
- Bob approves the access, and SmartGrocer receives an Authorization Code.
- SmartGrocer exchanges the code for an Access Token.
- SmartGrocer uses the token to make an API Request to process the payment.
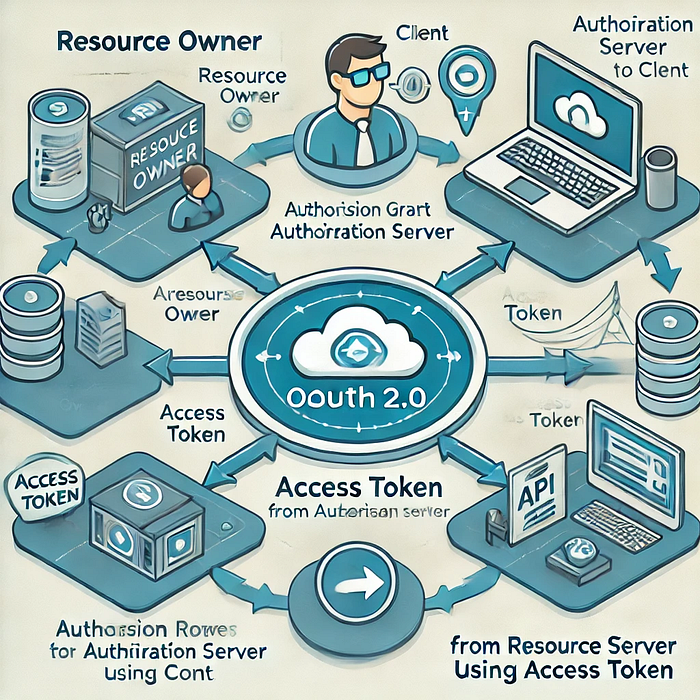
Securing RESTful APIs with OAuth 2.0 and Spring Security is essential for protecting data and ensuring authorized access. Combining real-world scenarios with technical insights provides a clearer understanding of the implementation process.
Key Takeaways:
- OAuth 2.0 enables secure, token-based access to APIs.
- Spring Security simplifies integration in Spring Boot applications.
- Best practices and real-world scenarios enhance both security and usability.
Find us
linkedin Shant Khayalian
Facebook Balian’s
X-platform Balian’s
web Balian’s
Youtube Balian’s
#OAuth2 #SpringSecurity #API #APISecurity #SpringBoot #Cybersecurity #RESTAPI #SecureCoding #JWT #JavaDeveloper