Best Practices Implementing Multi-Tenancy in Spring Boot SaaS
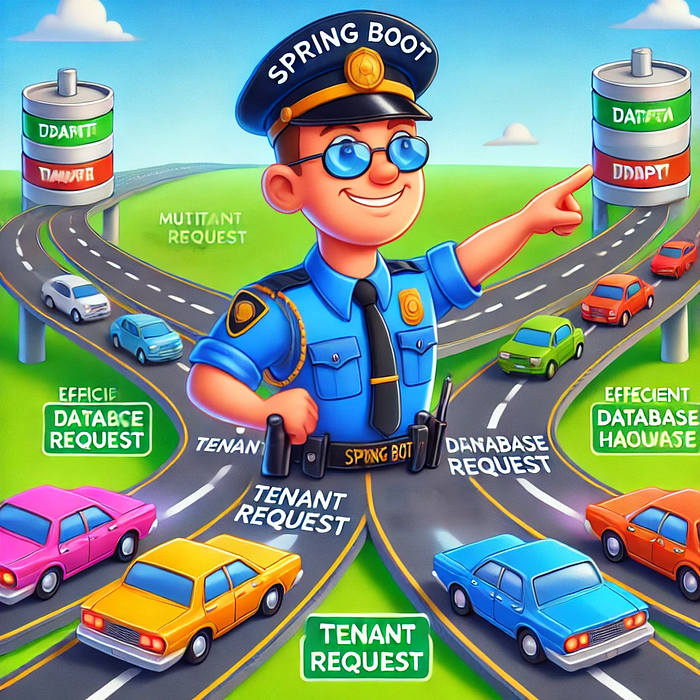
Views: 8
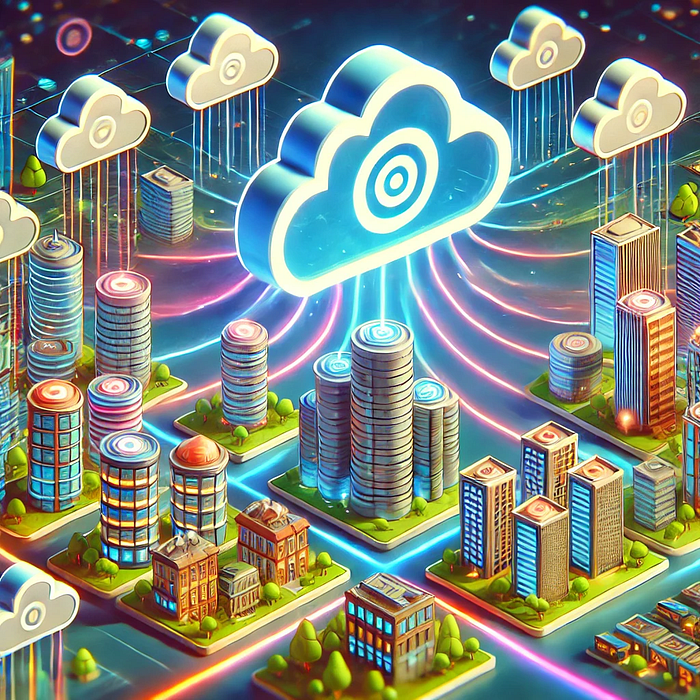
Best Practices for Implementing Multi-Tenancy in Spring Boot SaaS Apps
Let’s explore how to architect powerful, future-ready multi-tenant applications with Spring Boot.
What is Multi-Tenancy? Why Does It Matter?
Multi-tenancy refers to a software architecture where a single application instance serves multiple customers (tenants), ensuring that each tenant’s data remains isolated and secure.
Real-life analogy:
- Single-Tenant Model: Like building one house per family.
- Multi-Tenant Model: Like constructing an apartment complex where every family has its own unit, but they share infrastructure like plumbing and electricity.
Why It Matters:
✔️ Reduces operational costs
✔️ Simplifies maintenance
✔️ Enhances scalability
✔️ Streamlines resource usage
Real-Life Example: Apartment Complex vs. Single Houses
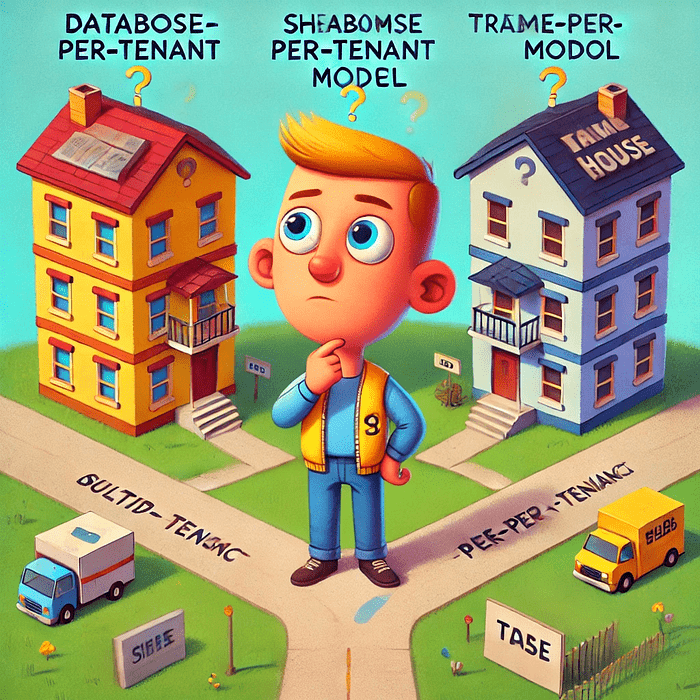
Imagine you’re a real estate developer:
- Single-Tenant Model → You build one house per family (One server/app per customer).
- Multi-Tenant Model → You build a large apartment complex where each tenant has their own private unit but shares the same building infrastructure (shared database, shared resources).
✔ Multi-tenancy saves costs, optimizes resources, and simplifies maintenance!
Choosing the Right Multi-Tenancy Strategy
Choosing the correct multi-tenancy model is crucial to your app’s success. Let’s unpack the three most popular strategies:
Database-per-Tenant (Isolated Model)
Each tenant gets a separate database.
Pros:
✔️ Highest data isolation and security
✔️ Ideal for enterprise-level clients
Cons:
✗ Expensive to manage
✗ Difficult to scale with thousands of tenants
Spring Boot Configuration:
spring:
datasource:
url: jdbc:mysql://tenant_database_url
username: tenant_user
password: tenant_password
Schema-per-Tenant (Moderate Isolation)
Each tenant uses a unique schema within a shared database.
Pros:
✔️ Balanced isolation and scalability
Cons:
✗ Complex schema management, especially with migrations
Spring Boot Example:
public String resolveTenantSchema(String tenantId) {
return "tenant_" + tenantId;
}
Shared Database, Table-per-Tenant (Light Isolation)
A single database and schema; tables include a tenant_id
field.
Pros:
✔️ Highly scalable for thousands of tenants
Cons:
✗ Increased query complexity
✗ Possible performance bottlenecks
Spring Boot Entity Example:
@Entity
public class Order {
@Column(name = "tenant_id")
private String tenantId;
}
Implementing Multi-Tenancy in Spring Boot
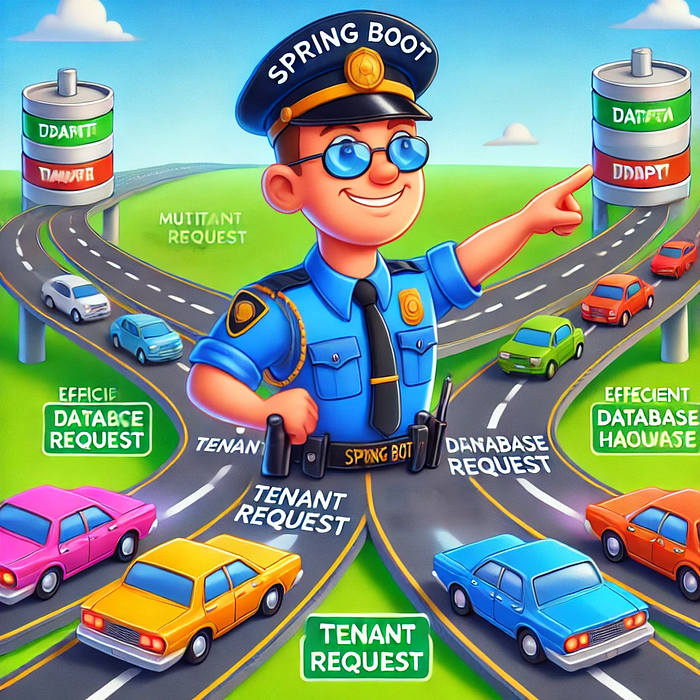
Step 1: Identify the Tenant
You can detect tenants from:
- HTTP headers
- Subdomain parsing (e.g.,
tenant1.myapp.com
) - JWT Token claims
Example:
URL → https://tenant1.myapp.com/orders
→ Tenant ID = tenant1
Step 2: Configure Dynamic DataSource Routing
Use a routing DataSource to switch tenants dynamically.
public class TenantRoutingDataSource extends AbstractRoutingDataSource {
@Override
protected Object determineCurrentLookupKey() {
return TenantContext.getCurrentTenant();
}
}
✔️ This ensures database switching at runtime.
Step 3: Use Hibernate Filters
To achieve tenant-specific data isolation:
@FilterDef(name = "tenantFilter", parameters = @ParamDef(name = "tenantId", type = "string"))
@Filter(name = "tenantFilter", condition = "tenant_id = :tenantId")
public class Order { ... }
✔️ This automatically filters tenant-specific data during queries.
Security Considerations for Multi-Tenant Apps
Security is non-negotiable in a multi-tenant environment.
Best Practices:
- Use auditing and logging for traceability
- Enforce strict tenant isolation at database, application, and UI layers
- Implement OAuth2 or JWT token-based authentication
- Apply rate-limiting to prevent abusive tenant behavior
Scaling Multi-Tenant SaaS Apps
Scaling is essential to handle growing tenant bases.
Key Techniques:
- Employ load balancers smartly to distribute tenant traffic
- Deploy Kubernetes for automated scaling
- Implement database sharding for managing data overflow
- Use Redis or Memcached for caching tenant metadata
Which Multi-Tenancy Model is Best for You?
Criteria | Database-per-Tenant | Schema-per-Tenant | Table-per-Tenant |
---|---|---|---|
Security | High | Medium | Low |
Scalability | Low | Medium | High |
Complexity | High | Medium | High |
Cost | High | Medium | Low |
Best Practices for Implementing Multi-Tenancy in Spring Boot SaaS Apps require balancing security, performance, and scalability. Selecting the right model and implementing dynamic tenant resolution will not only future-proof your SaaS application but also create delightful, secure user experiences.
Investing in a robust multi-tenant architecture today ensures your SaaS solution grows gracefully tomorrow.
FAQs
What is the easiest multi-tenancy strategy to implement?
Schema-per-tenant is usually the best compromise between ease of implementation and scalability.
How do you secure tenant data in a shared database model?
By enforcing row-level security policies and using Hibernate filters.
Can I migrate from one multi-tenancy model to another?
Yes, but it can be complex and may involve data migration scripts and downtime.
How does multi-tenancy affect performance?
Poorly implemented multi-tenancy can lead to query bloat; caching and indexing are crucial.
Is Kubernetes necessary for scaling multi-tenant apps?
While not mandatory, Kubernetes greatly simplifies scaling and management.
Which databases are best for multi-tenant SaaS?
PostgreSQL, MySQL, and MongoDB are popular choices due to their flexibility.
Helpful Sources
- Link to: Hibernate Filters Official Documentation
- Link to: Kubernetes Official Documentation
Also Check
- Link to: Handling 1 Million Messages per Second
- Link to: Observability Jaeger Spring Boot: 7 Easy Steps for Full Tracing
Find us
Balian’s Blogs Balian’s
linkedin Shant Khayalian
Facebook Balian’s
X-platform Balian’s
web Balian’s
Youtube Balian’s
#springboot #multitenancy #saas #microservices #softwarearchitecture #cloudnative #scalability #devops #databasearchitecture