Kafka Consumer Patterns in Spring Boot: Best Practices for High-Performance
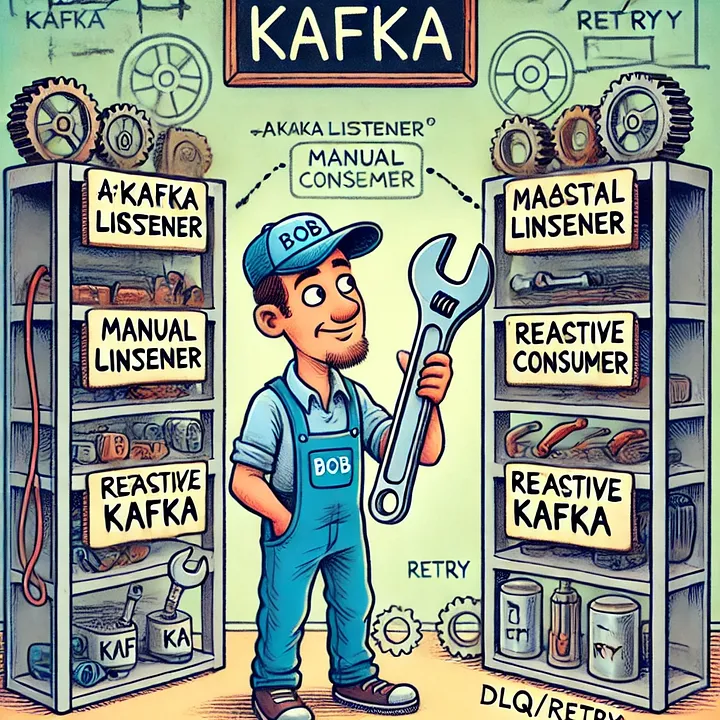

What This Guide Covers
Kafka is powerful. But how you consume messages can either throttle or turbocharge your system.
In this deep-dive, we’ll cover:
- 3 Kafka consumption patterns:
@KafkaListener
, manual polling, and reactive Kafka - Advanced considerations: throughput, backpressure, retries, and error recovery
- Real-world metaphors with Bob (our everyman hero)
- Illustrated cartoon visuals to solidify each mental model
- Retry and Dead Letter logic for production-grade reliability
1. Meet Bob
Bob isn’t a developer. He’s just a regular guy who navigates life like we all do:
- Doctor’s appointments
- Airports and travel
- Mobile check-ins
- Burrito emergencies
We’ll use Bob’s everyday scenarios as mental models to understand Kafka consumer behavior.
Because, believe it or not, Kafka is everywhere — even in Bob’s daily life.
2. @KafkaListener
: Bob at the Doctor’s Office

Bob walks into his local clinic. He:
- Takes a number
- Waits in a crowded room
- Gets called one by one
- Sees the doctor only when it’s his turn
This is exactly how @KafkaListener
works.
Code Example
@KafkaListener(topics = “invoices”, groupId = “billing”) public void listen(String message) { processMessage(message); // Simulates Bob’s checkup }Technical Breakdown

Bob’s Verdict: “It works, but man… that waiting room was painful.”

4. Reactive Kafka: Bob Checks In Online
Now Bob’s just flexing.
From his phone, he:
- Checks in online
- Gets a mobile boarding pass
- Arrives, scans, and walks right in
No threads. No blocking. Everything is event-driven and backpressure-aware.
Code Example
KafkaReceiver.create(receiverOptions) .receive() .concatMap(record -> Mono.fromRunnable(() -> { process(record.value()); record.receiverOffset().acknowledge(); })) .subscribe();Technical Breakdown

Bob’s Verdict: “I checked in while brushing my teeth. Welcome to the future.”

Retry & Dead Letter Queues: Bob’s Burrito Catastrophe
Bob orders a burrito. But:
- It’s too spicy
- It’s delivered to the wrong address
- His card gets declined
Each failure is retried — but after 3 tries, the burrito is trashed and he gets a refund.
In Kafka, we need the same logic: retry a few times, then move the message to a Dead Letter Topic (DLT).