My Top 10 Java Utility Functions You’ll Want to Steal
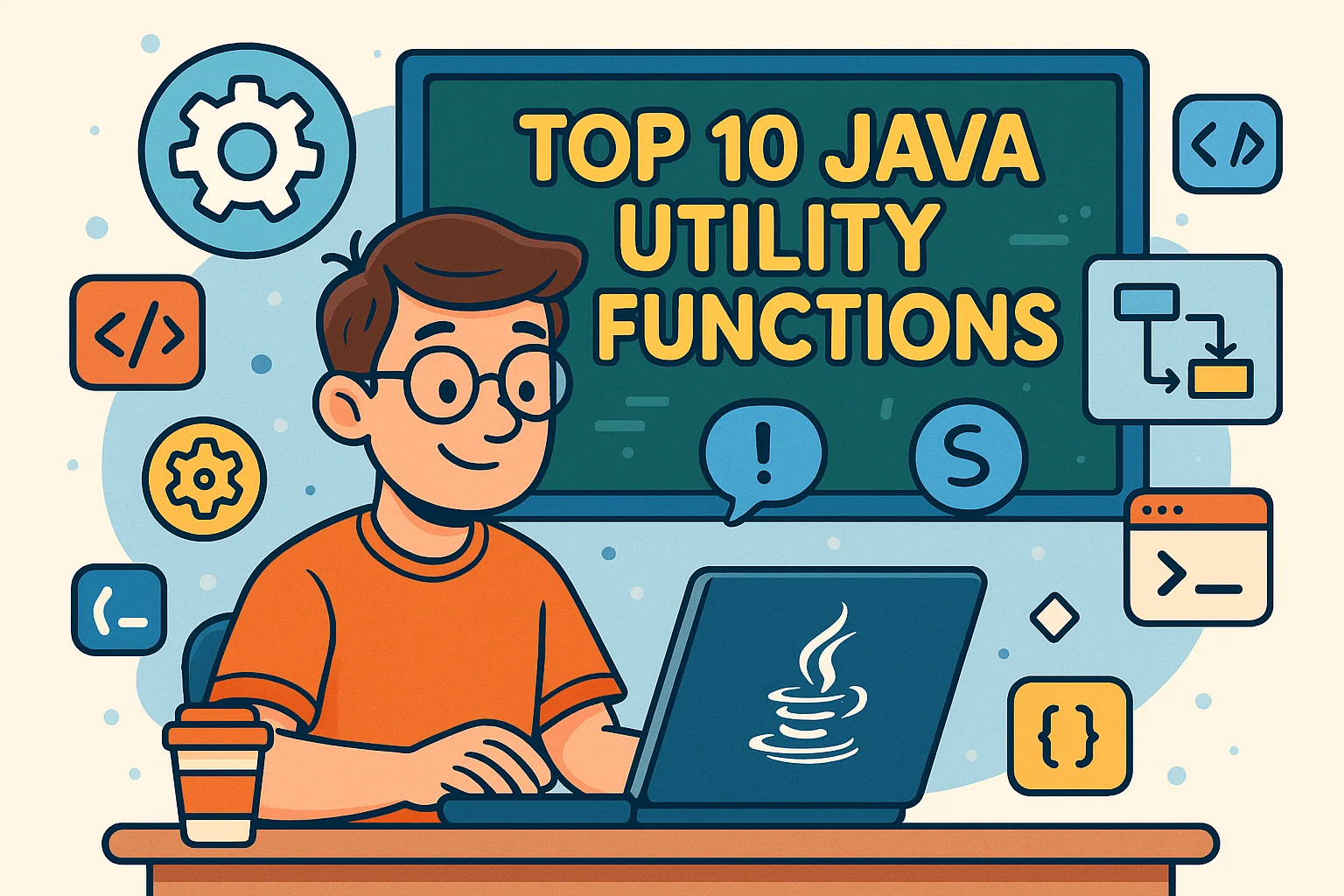
Unlocking Java’s hidden gems with Bob’s everyday adventures.
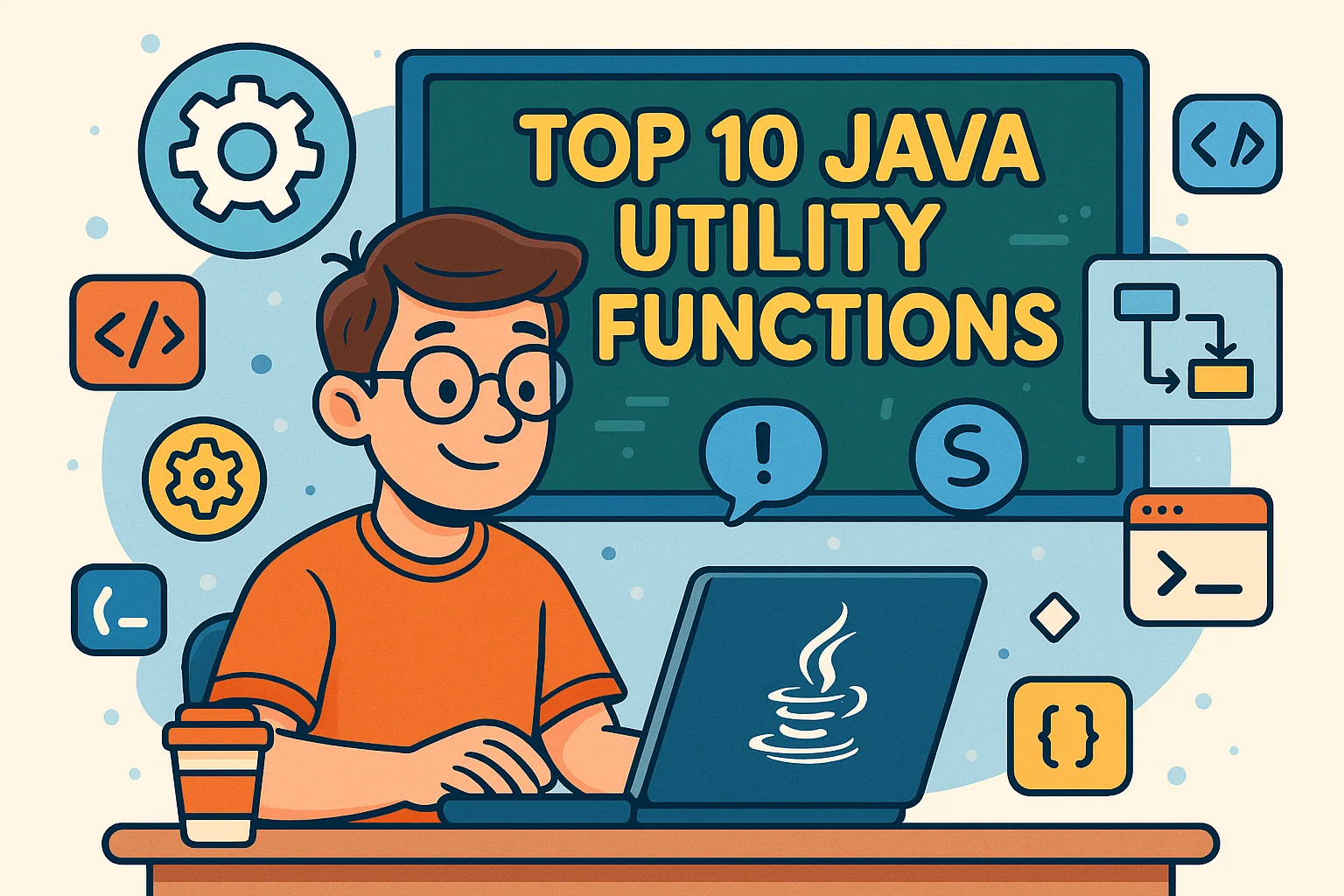
The Power of Utility Functions
In Java development, utility functions are like the Swiss Army knives of coding — they provide reusable solutions to common problems, enhancing code readability and maintainability. Typically housed in utility classes (often with names ending in Utils
), these static methods help avoid code duplication and promote best practices.
Your Everyday Java Developer
To make our exploration relatable, let’s follow Bob, a Java developer navigating daily coding challenges. Through his experiences, we’ll see how utility functions can simplify tasks and improve code efficiency.
Top 10 Java Utility Functions
1. isNullOrBlank(String input)
Purpose: Checks if a string is null
or contains only whitespace.
public static boolean isNullOrBlank(String input) {
return input == null || input.trim().isEmpty();
}
Bob’s Scenario: Bob is validating user input from a form. Using isNullOrBlank
, he ensures that fields aren’t left empty or filled with just spaces.
2. capitalize(String input)
Purpose: Capitalizes the first character of the string.
public static String capitalize(String input) {
if (isNullOrBlank(input)) return input;
return input.substring(0, 1).toUpperCase() + input.substring(1);
}
Bob’s Scenario: While displaying user names, Bob wants to ensure they start with a capital letter for consistency.
3. join(List<String> list, String delimiter)
Purpose: Joins a list of strings into a single string with a specified delimiter.
public static String join(List<String> list, String delimiter) {
return String.join(delimiter, list);
}
Bob’s Scenario: Bob needs to display a list of selected items as a comma-separated string.
4. reverse(String input)
Purpose: Reverses the given string.
public static String reverse(String input) {
return new StringBuilder(input).reverse().toString();
}
Bob’s Scenario: Implementing a feature to check for palindromes, Bob uses reverse
to compare strings.
5. isPalindrome(String input)
Purpose: Checks if a string reads the same backward as forward.
public static boolean isPalindrome(String input) {
if (input == null) return false;
String clean = input.replaceAll("\\s+", "").toLowerCase();
return clean.equals(reverse(clean));
}
Bob’s Scenario: Bob adds a fun feature to detect palindromic phrases entered by users.
6. safeParseInt(String input)
Purpose: Safely parses a string to an integer, returning 0 if parsing fails.
public static int safeParseInt(String input) {
try {
return Integer.parseInt(input);
} catch (NumberFormatException e) {
return 0;
}
}
Bob’s Scenario: Processing form inputs, Bob uses safeParseInt
to handle optional numeric fields gracefully.
7. distinctByKey(Function<T, ?> keyExtractor)
Purpose: Filters a stream to ensure distinct elements based on a key.
public static <T> Predicate<T> distinctByKey(Function<? super T, ?> keyExtractor) {
Set<Object> seen = ConcurrentHashMap.newKeySet();
return t -> seen.add(keyExtractor.apply(t));
}
Bob’s Scenario: Bob processes a list of users and wants to remove duplicates based on email addresses.
8. retry(Runnable task, int attempts)
Purpose: Retries a task a specified number of times if it fails.
public static void retry(Runnable task, int attempts) {
for (int i = 0; i < attempts; i++) {
try {
task.run();
return;
} catch (Exception e) {
if (i == attempts - 1) throw e;
}
}
}
Bob’s Scenario: Bob implements a network call that occasionally fails; using retry
, he attempts the call multiple times before giving up.
9. timeExecution(Runnable task)
Purpose: Measures and logs the execution time of a task.
public static void timeExecution(Runnable task) {
long start = System.currentTimeMillis();
task.run();
long end = System.currentTimeMillis();
System.out.println("Execution time: " + (end - start) + " ms");
}
Bob’s Scenario: Optimizing performance, Bob uses timeExecution
to identify slow-running methods.
10. memoize(Function<T, R> function)
Purpose: Caches the results of expensive function calls.
public static <T, R> Function<T, R> memoize(Function<T, R> function) {
Map<T, R> cache = new ConcurrentHashMap<>();
return input -> cache.computeIfAbsent(input, function);
}
Bob’s Scenario: Bob optimizes a recursive computation by caching results to avoid redundant processing.
Utility functions are invaluable tools in a Java developer’s toolkit. By abstracting common tasks into reusable methods, they promote cleaner, more maintainable code. Through Bob’s experiences, we’ve seen practical applications of these functions, demonstrating their relevance in everyday coding scenarios.
FAQ
What is a utility function in Java?
A utility function in Java is a static method that performs a commonly used, reusable operation — often unrelated to any specific object state.
Examples:
- String manipulation (
capitalize
,reverse
) - Data conversion (
safeParseInt
) - Collections handling (
join
,distinctByKey
)
They typically reside in utility classes like StringUtils
, DateUtils
, or AppUtils
.
Key Trait: They don’t depend on instance variables and are usually declared static
.
What is the use of a utility class in Java?
A utility class groups multiple utility functions together for:
- Code reuse
- Clean and DRY code
- Easy testing and debugging
- Faster development
Example: A MathUtils
class might offer static methods like add(a, b)
, isPrime(n)
, and factorial(n)
.
How to import a utility class in Java?
Assuming you have a utility class named AppUtils
in the package com.example.utils
, you import it like this:
import com.example.utils.AppUtils;
Or to import all utility classes from a package:
import com.example.utils.*;
Note: Since utility methods are static
, you can use static imports to call them directly without the class name:
import static com.example.utils.StringUtils.capitalize;
String name = capitalize("bob"); // no need to call StringUtils.capitalize()
How to make a Java utility class?
Here’s a pattern you should follow to build a utility class:
public final class StringUtils {
// Private constructor to prevent instantiation
private StringUtils() {
throw new UnsupportedOperationException("Utility class");
}
public static boolean isNullOrBlank(String input) {
return input == null || input.trim().isEmpty();
}
public static String capitalize(String input) {
if (input == null || input.isEmpty()) return input;
return input.substring(0, 1).toUpperCase() + input.substring(1);
}
}
Tips for utility classes:
- Use
final
to prevent subclassing - Include a private constructor
- Keep methods static
- Avoid holding state or instance variables
Find us
Balian’s Blogs Balian’s
linkedin Shant Khayalian
Facebook Balian’s
X-platform Balian’s
web Balian’s
Youtube Balian’s
#Java #UtilityFunctions #CodeReuse #JavaBestPractices #CleanCode #JavaDevelopment
k9rh57